When it comes to web app development, there are often many good ways to build an app. Others are better. In ArcGIS, starting with a web map built in Map Viewer is always best.
The ArcGIS Maps SDK for JavaScript (JS Maps SDK) provides you with a wide range of APIs, components, and resources that enable you to build web mapping applications for a variety of workflows. Building a map from scratch using the WebMap class is good because it keeps you from having to do graphics development yourself.
However, there is a better way. While the JS Maps SDK boasts developer-friendly APIs and hundreds of samples that demonstrate how to build apps from the ground up using only JavaScript, CSS, and HTML, there is an often-overlooked tool that can significantly ease your web app development—Map Viewer in ArcGIS Online or your ArcGIS Enterprise portal.
In this article, I’ll build an application that explores the number of people visiting U.S. national parks from year to year. In the process, I’ll demonstrate how using Map Viewer in your development workflow is the best approach to take when creating a web mapping application. I’ll also compare it with other common approaches that are good, but don’t carry the same benefits.

Map Viewer
Map Viewer’s name doesn’t adequately portray the full power of its capabilities. In addition to allowing you to view web maps, Map Viewer gives you tools to configure a vast array of properties for web maps and layers with a simplified, intuitive UI. These configurable properties include renderers, popups, labels, base maps, tables, and charts just to name a few.
For example, I’ve created this web map that explores the number of visitors to U.S. national parks in 2023. The size of each symbol corresponds to the number of visitors to each park and the color indicates how the number of visitors changed from the previous year.
Each of the following images shows a different configuration panel used to create the style (or renderer), labels, and popups in this map.



Once configured, layers and maps can be saved as portal items. A portal item is a JSON-based file hosted on ArcGIS Online or your Enterprise portal instance. As detailed below, items can be reused and shared with others.
Portal items have a unique itemId
that facilitates loading the corresponding web maps into any ArcGIS web application.
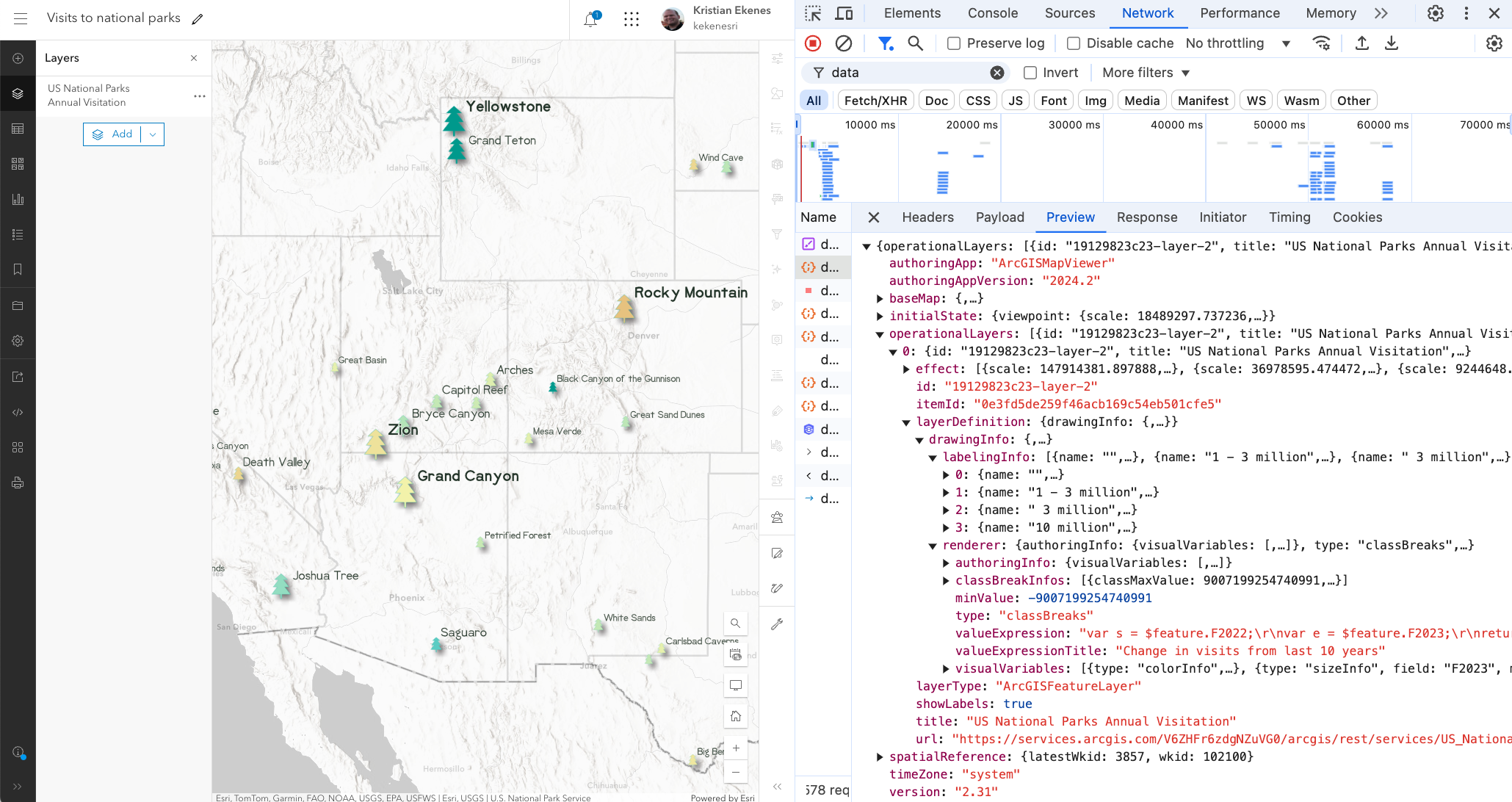
So, we have an app that lets you create a map. How does this benefit me, as a developer?
Benefits for developers
Leveraging Map Viewer to save web maps and layers as portal items has several benefits for development workflows.
- No code app development
- Low code app development
- Efficient teamwork
- Flexibility for changes
- Higher capacity to do more
Let’s dive into each of these in the context of the web map created above.
No code app development
In many cases, you don’t need to write a single line of code to deploy a web app based on a map you configure in Map Viewer. Esri provides many configurable apps, covering a variety of common use cases, that allow you to load the map in a predefined layout you can easily modify according to your needs.
Within Map Viewer, simply click the “create app” action and select one of the configurable app options: Instant Apps, Experience Builder, ArcGIS StoryMaps, or Dashboards.

In the example below, I used the “Insets” Instant App because a lot of the data in my national parks app is located in Alaska and Hawaii. Check it out.

This workflow doesn’t require any app development experience and makes maintaining apps easy.
Low code app development
You can load maps created in Map Viewer in custom low-code applications built with the JS Maps SDK. Not all app designs and use cases are satisfied by out-of-the-box apps. This is where you need to put on your developer hat and use the JS Maps SDK. Fortunately, configuring a web map in Map Viewer greatly simplifies the code required to render the map in a simple web app.
Load a web map with map components
For example, I built the following application from scratch by loading the web map configured above to an app built with the JS Maps SDK.

Here’s the entirety of the code required for this app:
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="initial-scale=1, maximum-scale=1,user-scalable=no" />
<title>National Park Visits</title>
<style>
html,
body {
padding: 0;
margin: 0;
height: 100%;
width: 100%;
}
</style>
<!-- Load Calcite components from CDN -->
<link rel="stylesheet" type="text/css" href="https://js.arcgis.com/calcite-components/2.13.2/calcite.css" />
<script type="module" src="https://js.arcgis.com/calcite-components/2.13.2/calcite.esm.js"></script>
<!-- Load the ArcGIS Maps SDK for JavaScript from CDN -->
<link rel="stylesheet" href="https://js.arcgis.com/4.31/esri/themes/light/main.css" />
<script src="https://js.arcgis.com/4.31/"></script>
<!-- Load Map components from CDN-->
<script type="module" src="https://js.arcgis.com/map-components/4.31/arcgis-map-components.esm.js"></script>
</head>
<body>
<arcgis-map item-id="8aa8e543e8e0446e8e2937e2b743b9f0">
<arcgis-zoom position="top-left"></arcgis-zoom>
<arcgis-expand expanded position="top-left">
<arcgis-legend></arcgis-legend>
</arcgis-expand>
</arcgis-map>
</body>
</html>
The app is a mere 38 lines long!
Also, note that by creating the app using map components and loading a web map configured in Map Viewer, I didn’t have to write a single line of JavaScript. It is pure HTML and CSS, even for adding other map components, like a legend.
In this case, the configuration of the map in Map Viewer takes the bulk of the time to create the app, whereas the time needed for actual app development was trivial.
Build the same app without a web map or map components
In comparison to the previous workflow, I built the same app without Map Viewer or map components.
This involves using JavaScript to configure a layer with a popup containing a line chart, data-driven labels, renderer, and effects and adding it to map with a basemap containing a hillshade layer blended with three vector tile layers.

To create this, I needed to write 775 lines of JavaScript 😬! Scroll through the code here to get a sense of what that looks like.
The APIs used to create this app are good and have been carefully designed to be intuitive. However, setting this many configurations using only JavaScript can be a tedious, time-consuming game of trial and error. How do I know which stops and break points to set in my renderer? Or what about picking suitable colors that go well with my basemap? How do I design good labels with an appropriate font size?
Map Viewer makes finding the answers to these questions trivial. In fact, I find the process for exploring different visualizations and configurations enjoyable, whereas it would be tedious, prone to silly errors, and frustrating if I spent time guessing values in JavaScript and refreshing and rebuilding my app just to see the changes. Map Viewer’s components are highly interactive and performant, giving you instantaneous feedback throughout the configuration process.
Once you’re done with your map, simply save it and paste the item ID of the web map into the item-id
property of the arcgis-map
component in your custom application.
Efficient teamwork
Map Viewer facilitates more efficient teamwork in the app development process. Because configuring a map in Map Viewer uses an intuitive user interface, you don’t actually need development experience at all to be part of the web app creating process. Therefore, the bulk of the time spent in the app development cycle doesn’t need to be spent by an app developer.
If you have an in-house GIS specialist, they can do the map configuration on their own and provide you—the developer—with the item id so you don’t need to worry about any of the map configuration.
This also saves time when team dynamics involve a lot of change requests between developers and cartographic designers. The GIS specialist can maintain control of the cartography of the map, while removing extra burden on the developer.
Flexibility for changes
Map Viewer also makes it easy to deploy changes to maps that may be consumed by many web and mobile applications. Data changes, either due to edits or live feeds, may necessitate changes in a web map’s configuration. For example, in the National Park Visitation map, the data will eventually be updated with 2024 numbers. When that happens, the web map popup, renderer, and labels will need to be updated to point to the field containing numbers for 2024 visits.
If all the configurations were done in JavaScript, the app code would need to be updated, rebuilt, and re-deployed with even the most minor changes. Imagine building 10 apps, consuming the same data, that also had to have consistent layer configurations, and being forced to copy and paste layer configurations 10 times all in JavaScript. Any change you make would have to be made in each of the apps.

If the configuration is done completely in the portal, then the app code doesn’t need to change because the associated item Id would remain constant. Any change to the portal item automatically gets picked up the next time the app is loaded. This makes the process for updating map and layer configurations more flexible and thus much more efficient.

Higher capacity to do more
Because layer configurations can be saved to the portal, you can reuse items in multiple apps (see image above) saving you development time and maintenance. This increased efficiency and flexibility in creating web apps naturally leads to an increased capacity to do more. You will have the time and ability to create and maintain more web apps than you could without the portal.
Conclusion
Building a mapping application using the powerful APIs available in the ArcGIS Maps SDK for JavaScript is good. Using the map components to reduce your JavaScript is a better approach. Starting with Map Viewer and saving your configurations to portal items is always the best approach. This will save you time, maintenance, and leave more time for focusing on core app functionality and interactivity.
Generally speaking, when building an app with the JS Maps SDK, I always suggest striving to write as few lines of code as possible. If you can get away with no code using an app builder, go for it!
Stay tuned for another article that will demonstrate how to add custom functionality to an app loading a web map in a JS Maps SDK application.
Article Discussion: