The first blog post of our series provided a comprehensive introduction to 3D object layers. They act as a system of record, allowing flexible, ad-hoc editing of 3D features directly on the web. You learned how to publish these layers, set sharing permissions, manage caching, and adhere to best practices. By leveraging 3D object layers within the ArcGIS Maps SDK for JavaScript, you can implement a variety of 3D object workflows in your web applications.
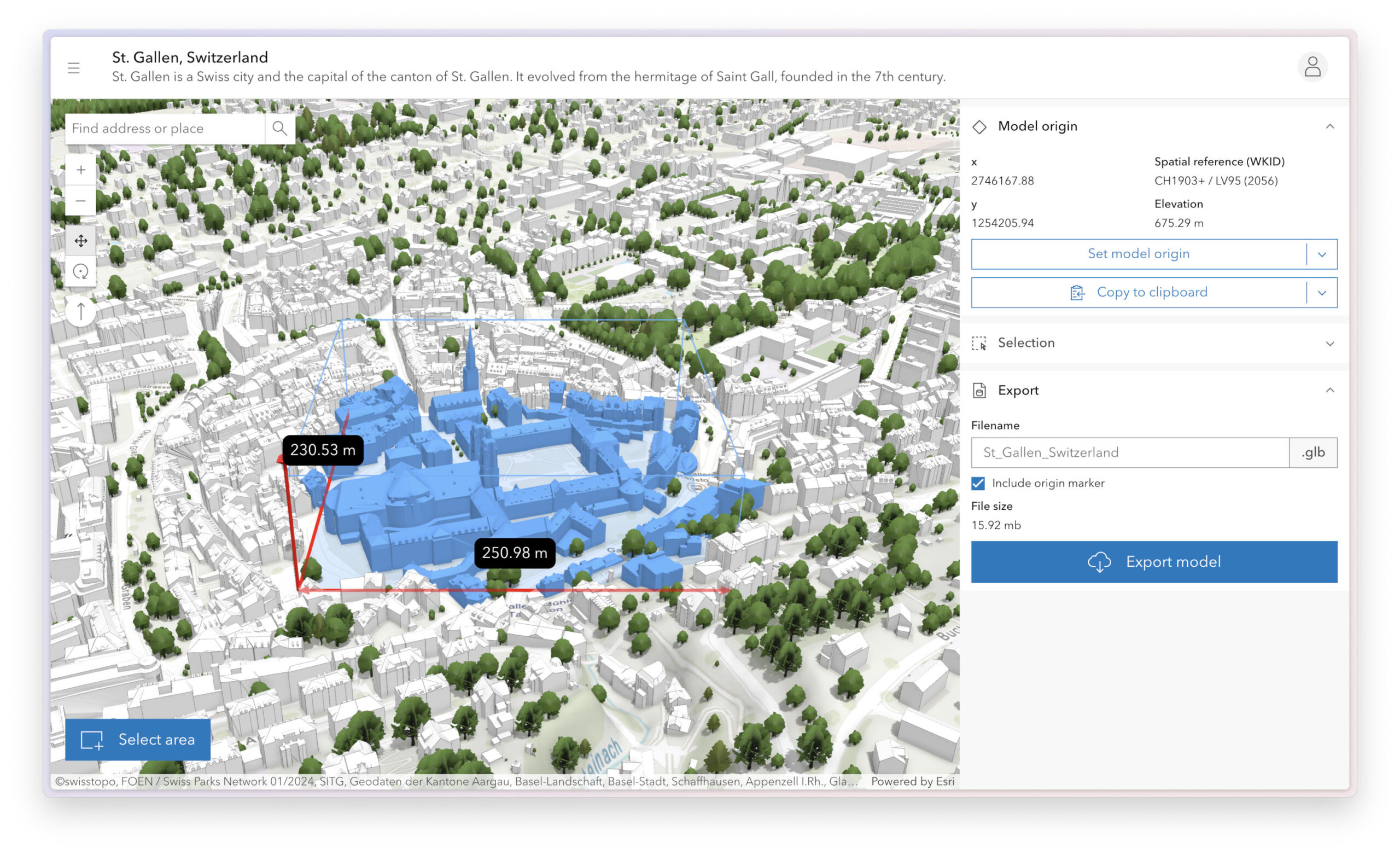
Utilizing the Editor widget—the built-in editing component—you can easily replicate the editing experience available in Scene Viewer. While this straightforward workflow will meet the needs of many users, some scenarios may require a more tailored approach. In such cases, you will need to learn how to integrate smaller specialized functions the API provides into larger functional units. This blog post will offer resources to assist you in achieving that.
This blog post has two primary objectives:
- Provide a quick tutorial on setting up your web app and recreating the Scene Viewer 3D object editing experience.
- Direct you to our new 3D object workflows in the SDK guide page. On this page, you can find comprehensive information for implementing your custom workflows.
By engaging with these resources, you will be well prepared to implement an advanced 3D object editing scenario similar to the one demonstrated in the SceneLayer Upload 3D Models and ApplyEdits sample from the JavaScript Maps SDK documentation.
3D object layer in the SDK
In June 2023, ArcGIS Maps SDK for JavaScript version 4.27 introduced 3D object editing functionality. Historically, the SceneLayer class has managed all subtypes of scene layers, including the 3D object type. This update seamlessly integrated editing capabilities into the SceneLayer class. The class automatically handles all interactions with the associated feature layer. This enables users to perform all 3D object editing tasks with just a SceneLayer instance.
Recreating Scene Viewer-like 3D object editing experience
Creating or loading a web scene in the JavaScript SDK requires just a few lines of code. The tutorials Display a Scene and Display a Web Scene on the ArcGIS Maps SDK for JavaScript website provide excellent guidance for this process. However, these introductory tutorials do not cover viewing modes and setting up spatial references for your use case, which is essential for real-world applications. Here, we address this gap.
Loading an existing web scene
When loading an existing web scene, it should already include a base map, an elevation layer, and the 3D object layer in the desired spatial reference. You learned how to create one in the first blog post, in the prerequisites of the Editing 3D Models in Scene Viewer chapter. The benefit of loading an already correctly set-up scene is that the correct spatial references will be loaded into your WebScene and the corresponding SceneView.
const view = new SceneView({
container: 'viewDiv',
map: new WebScene({
portalItem: {
// Replace with PortalItemID.
id: 'replaceMe',
},
}),
});
Creating a new web scene
When creating a new web scene, choose the viewing mode based on the available data.
Global scene: visualize 3D features on the globe
This assumes that our base maps, elevation, and other layers are available and published in the WGS 1984 or Web Mercator spatial reference. The 3D object layer should be published in the WGS 1984 spatial reference. Create a new global scene and use its default spatial reference.
Local scene: visualize 3D features on an approximated flat surface
This assumes our layers, base maps, elevation layers, and the 3D object layer are available and published in the desired projected coordinate system. Create a new local scene and specify the desired spatial reference.
In our example, we are using LV95, a PCS for Switzerland. The following code snippet creates an LV95 scene from scratch.
const scene = new WebScene({
basemap: new Basemap({
// LV95 Swiss Topographic (with Contours and Hillshade).
portalItem: { id: "03af6dc44c1e4c948eb87bbaef248f7a" },
}),
ground: {
layers: [
new ElevationLayer({
// LV95 Swiss Terrain 3D.
portalItem: { id: "0eab5ffc50da4cd2afe767c2f2851e30" },
}),
],
},
layers: [
new SceneLayer({
// Replace with 3D Object (Scene) Layer portal item ID.
portalItem: { id: "replaceMe" },
}),
],
});
Using the Editor widget
Using the Editor widget in ArcGIS Maps SDK for JavaScript allows you to replicate the Scene Viewer’s 3D object editing experience. To implement it, create or load a scene with a 3D object layer, instantiate a new Editor instance in your app, and add it to the user interface.
// Create an editor widget
const editor = new Editor({
view: view,
});
// Add it to the UI
view.ui.add(editor, {
position: 'top-right',
});
That’s all there’s to it! You can now edit 3D models like you can in Scene Viewer.
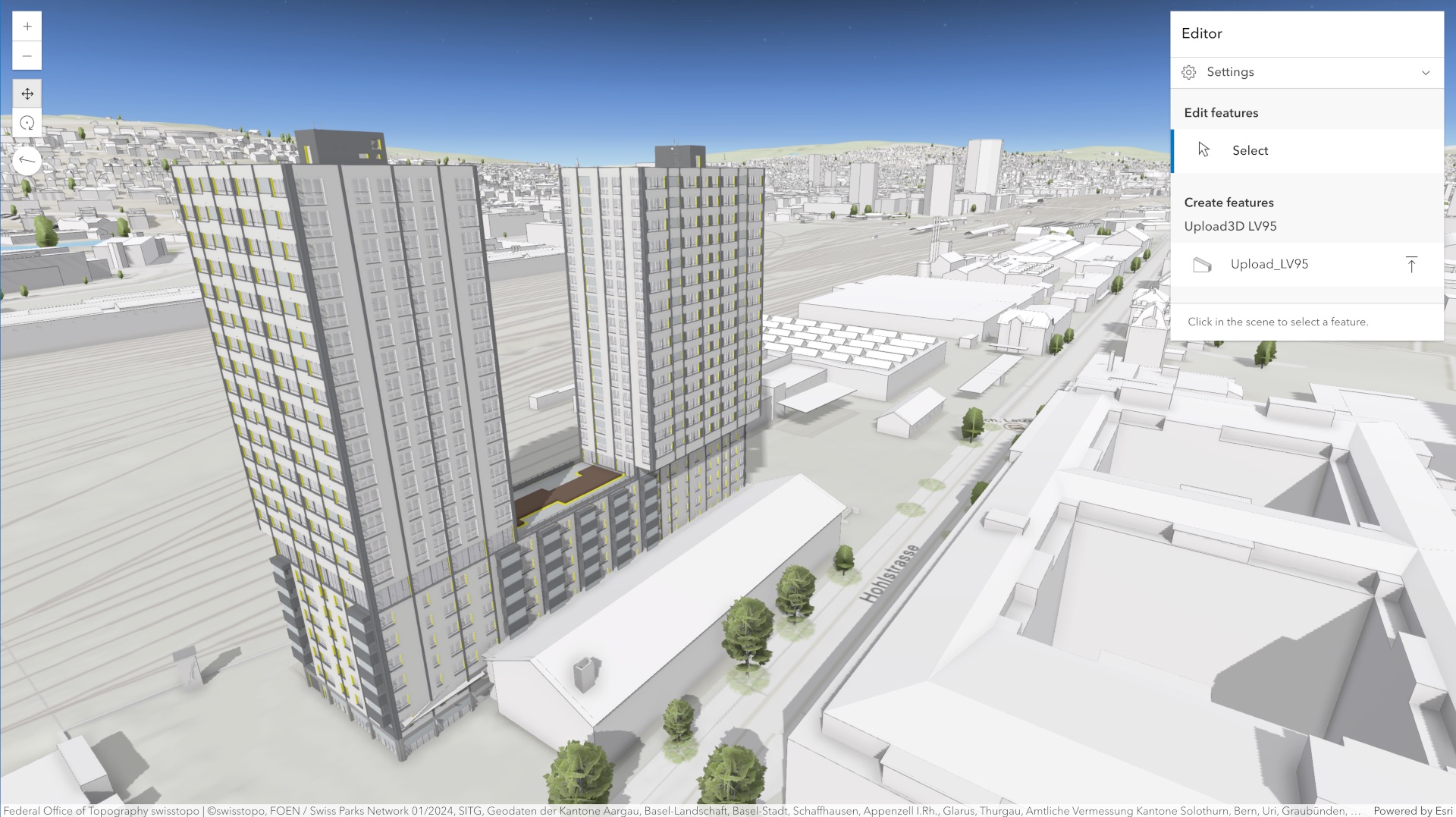
Introducing the “3D object workflows” guide page
For those looking to implement a truly customized 3D object editing experience, we encourage you to explore our new guide page on 3D object workflows in the JavaScript Maps SDK documentation. Similar to our first blog post, this guide is comprehensive and provides all the essential information you need. In addition to the topics covered in this blog post, you will find information on:
- 3D object layer implementation in a nutshell
- Format conversion
- Georeferencing
- Storage and updates
- Loading 3D files
- Displaying loaded meshes
- Placing and manipulating features with SketchViewModel
- Querying by features’ unique ID / Spatial querying
- Selecting 3D features with a mouse-click event and retrieving their geometry
- Updating and deleting features from the 3D object layer
What’s next?
With these resources, you will be well-equipped to implement an advanced 3D object editing scenario akin to the SceneLayer Upload 3D Models and ApplyEdits sample from the JavaScript Maps SDK documentation. The steps outlined at the end of that sample align with the functionalities discussed in this post and the guide. We highly encourage you to explore it and attempt to implement such an advanced scenario independently.
With this foundation, we hope you feel empowered to tackle any challenges as you develop innovative applications using 3D object layers and other Esri technologies. If there are specific topics you’d like us to explore in future posts, please don’t hesitate to reach out. Wishing you all the best on your journey!
Commenting is not enabled for this article.