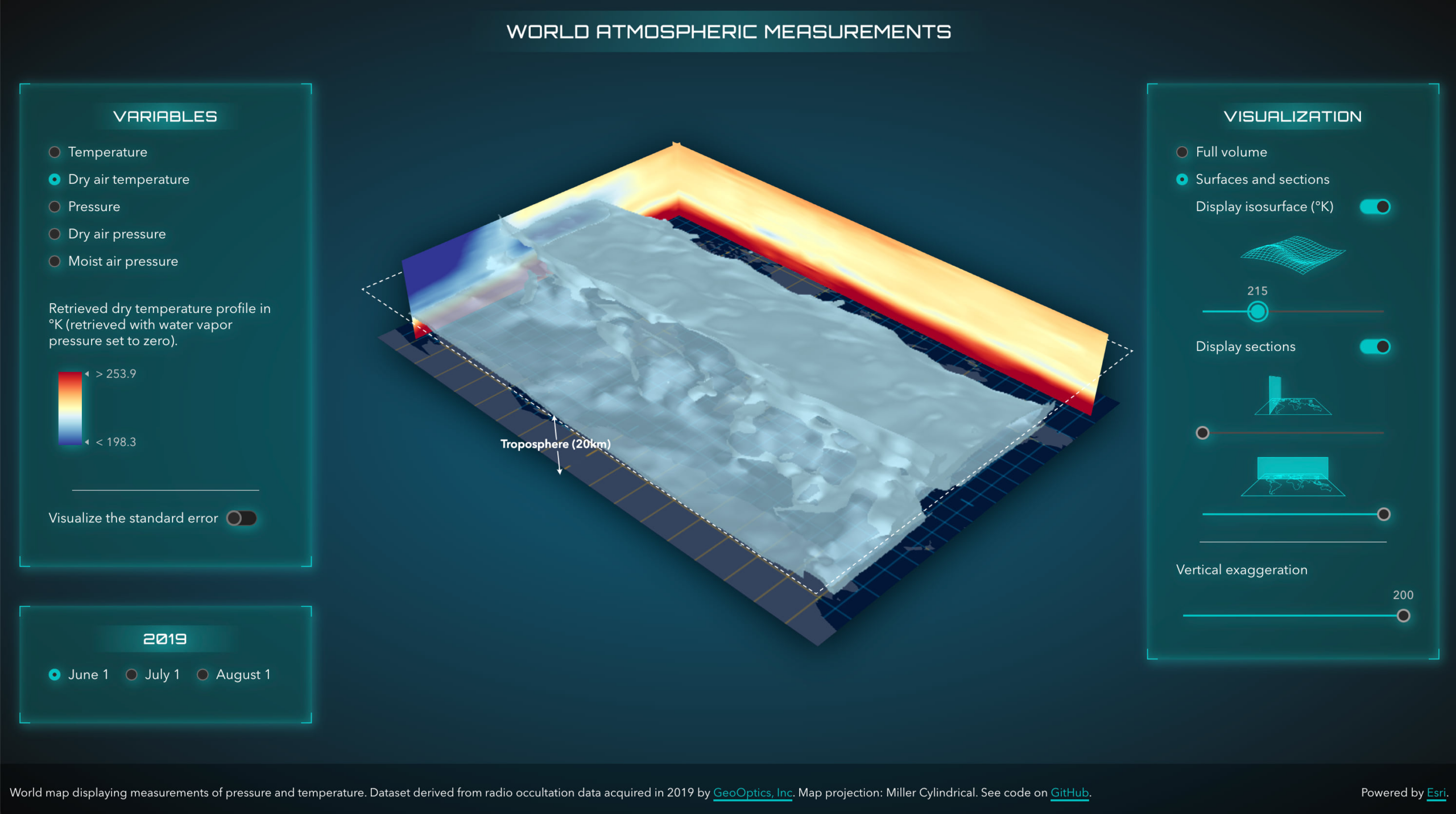
First of all, some definitions: atmospheric pressure is the force exerted on a surface by the air above as gravity pulls it to the Earth. The temperature at different levels of the Earth’s atmosphere is influenced by incoming solar radiation, humidity and of course by the altitude.
How can we measure temperature and pressure at such high altitudes?
The dataset is provided by GeoOptics, a company which specializes in radio occultations for weather forecasts. Each occultation event lasts less than a minute and produces a linear profile of between 350 and 400 observations from the top of the atmosphere to the bottom. The data are acquired from the altitude of 30km down to the ground level. The initial dataset contains
around 600 occultation profiles that we then use to interpolate the measurements into a 3D surface. The geostatistical interpolation method is called Empirical Bayesian Kriging 3D. Using GA Layer 3D to netCDF, we exported the 3D surface to a netCDF file that can then be viewed as a voxel layer.
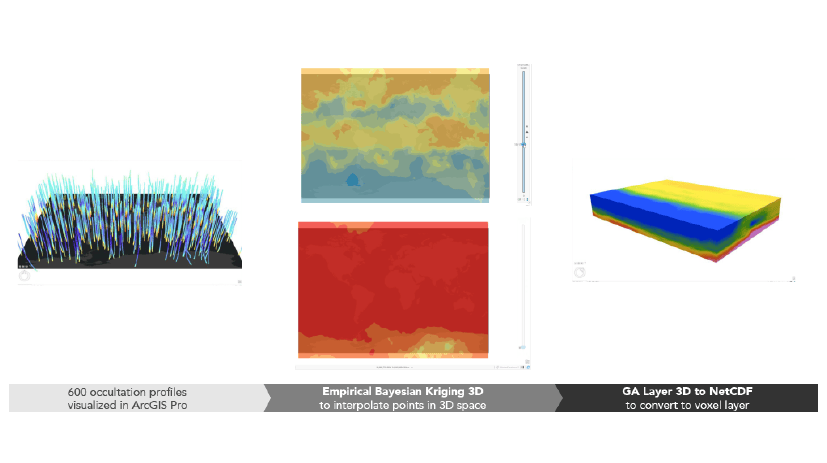
A voxel layer is a representation of multidimensional spatial and temporal information in a 3D volumetric visualization. In the Esri world this is a type of layer and we can publish it to ArcGIS Online and visualize in a browser. You can have a look at the voxel layer item here.
Exploring the voxel layer with ArcGIS API for JavaScript
Variables
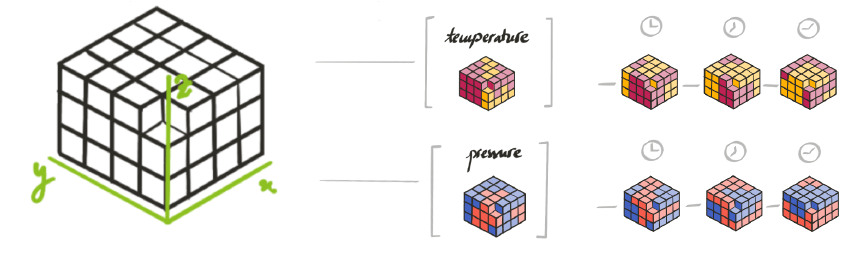
Within the ArcGIS API for JavaScript we can view information about variables by accessing the array voxelLayer.variables.
For example the temperature variable has this metadata:
{
description: "temperature_Prediction",
id: 10,
name: "temperature",
unit: "degree_K"
}
We can visualize temperature by setting the current variable id on the layer:
voxelLayer.currentVariableId = 10;
To change the time variable we set the time extent on view.timeExtent.
When we visualize them, we can compare the pressure and the temperature and we can see how pressure changes almost linearly with altitude, whereas temperature fluctuates much more because it doesn’t only depend on altitude, but also on solar radiation and humidity.
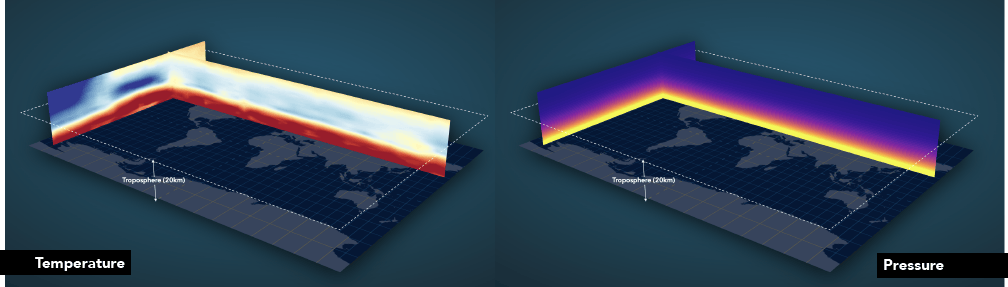
Rendering
volume
or surfaces
.Isosurfaces
voxelLayer.getVariableStyles(variableId).isosurfaces
.
const createIsosurface = value => {
const style = layer.getVariableStyles(selectedVariable.id);
const color = layer.getColorForContinuousDataValue(selectedVariable.id, value, false);
if (style) {
style.isosurfaces = [
{
value: value,
enabled: true,
color: {...color, a: 0.8},
// if the color is not locked, we can override it
colorLocked: false,
},
];
}
};
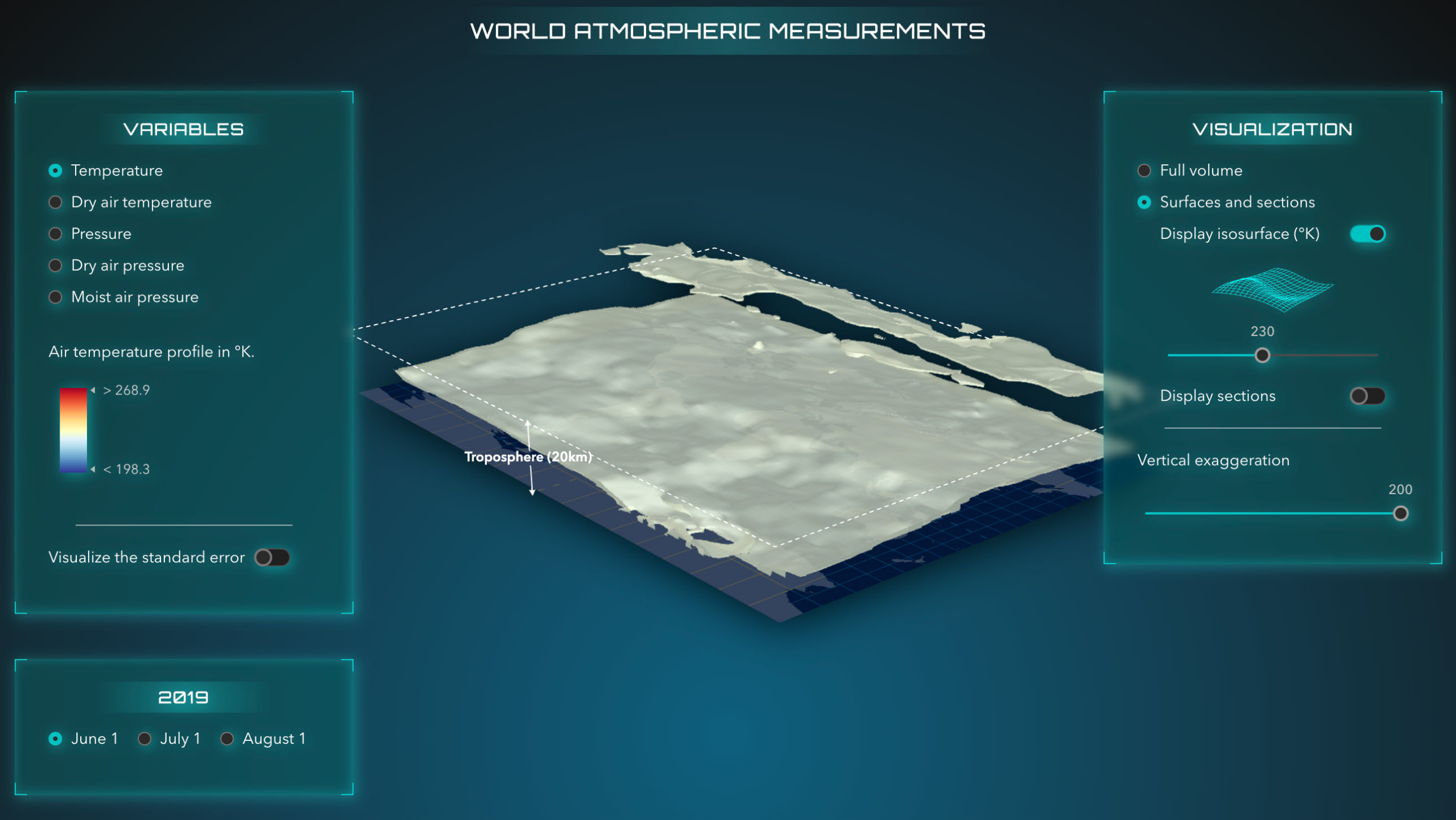
Sections
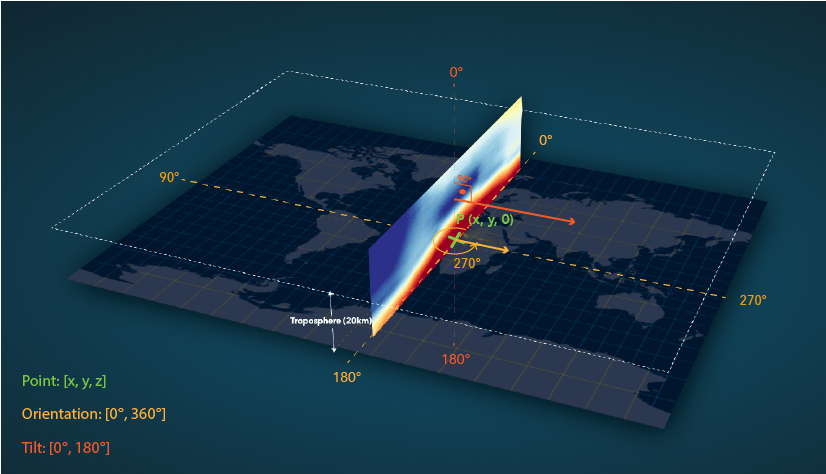
const dimensions = voxelLayer.getVolume().sizeInVoxels;
This is the code to generate a section that passes through the center of the voxel layer and is oriented from north to south like the image above:
layer.volumeStyles.getItemAt(0).dynamicSections = [
{
enabled: true,
orientation: 90,
tilt: 90,
point: [Math.floor(dimensions[0] / 2), 0, 0],
},
];
Note that the y and z coordinates of the point can have any value within the voxel space, with the given orientation and tilt, x is the coordinate that sets the position of the plane.
Slice the layer
const getSlice = height => {
return new VoxelSlice({
tilt: 0,
point: [0, 0, height],
});
};
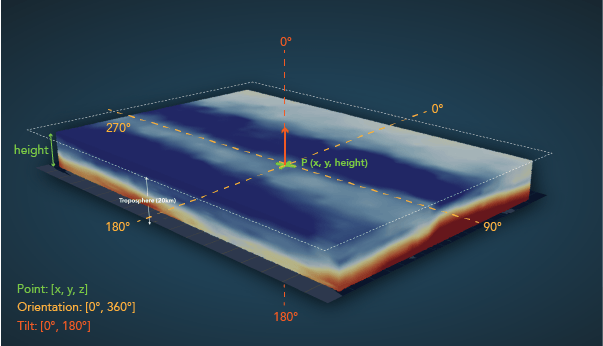
Article Discussion: